アプリ広告といえばGoogle AdMobの情報ばかりが出てきますが、今回はUnityで作ったゲームにUnity Adsを使ってみたので、導入方法を備忘録兼ねて書きたいと思います。
導入した広告タイプはインターシティシャル広告、バナー広告、リワード広告の3つです。
- MacBook Pro (13-inch, M1, 2020) macOS Monterey バージョン12.4
- Ads Package Version 3.7.5
- Unity 2021.3.11f Personal
- Android Studio Dolphin 2021.3.1
Unityの公式ドキュメントも参照してみてください。https://docs.unity.com/ads/CreatingUnityProjects.html
Unityスクリプト設定
GameIDを取得したら、Unityでスクリプトを書いてゲームに広告が出るようにする。
広告表示の基本の流れは 初期化→ロード→表示 です。
Unityでスクリプトファイルを作成
インターシティシャル広告、バナー広告、リワード広告すべてを表示したい場合は以下の4つのスクリプトファイルをUntiyのProject内で作成する。
AdsInitializer以外は必要なスクリプトのみ作成してもよい。
AdsInitializer
初期化のスクリプト。どの広告を表示する場合にも必要。
引用元: https://docs.unity.com/ads/InitializingTheUnitySDK.html
using UnityEngine;
using UnityEngine.Advertisements;
public class AdsInitializer : MonoBehaviour, IUnityAdsInitializationListener
{
[SerializeField] string _androidGameId;
[SerializeField] string _iOSGameId;
[SerializeField] bool _testMode = true;
private string _gameId;
void Awake()
{
InitializeAds();
}
public void InitializeAds()
{
_gameId = (Application.platform == RuntimePlatform.IPhonePlayer)
? _iOSGameId
: _androidGameId;
Advertisement.Initialize(_gameId, _testMode, this);
}
public void OnInitializationComplete()
{
Debug.Log("Unity Ads initialization complete.");
}
public void OnInitializationFailed(UnityAdsInitializationError error, string message)
{
Debug.Log($"Unity Ads Initialization Failed: {error.ToString()} - {message}");
}
}
InterstitialAd
インターシティシャル広告を表示するためのスクリプト。インターシティシャル広告はスキップできる広告のこと。
参考: https://docs.unity.com/ads/ImplementingBasicAdsUnity.html
using UnityEngine;
using UnityEngine.Advertisements;
public class InterstitialAd : MonoBehaviour, IUnityAdsLoadListener, IUnityAdsShowListener
{
[SerializeField] string _androidAdUnitId = "Interstitial_Android";
[SerializeField] string _iOsAdUnitId = "Interstitial_iOS";
string _adUnitId;
void Awake()
{
// Get the Ad Unit ID for the current platform:
_adUnitId = (Application.platform == RuntimePlatform.IPhonePlayer)
? _iOsAdUnitId
: _androidAdUnitId;
}
void Start()
{
LoadAd(); //追加
}
// Load content to the Ad Unit:
public void LoadAd()
{
// IMPORTANT! Only load content AFTER initialization (in this example, initialization is handled in a different script).
Debug.Log("Loading Ad: " + _adUnitId);
Advertisement.Load(_adUnitId, this);
}
// Show the loaded content in the Ad Unit:
public void ShowAd()
{
// Note that if the ad content wasn't previously loaded, this method will fail
Debug.Log("Showing Ad: " + _adUnitId);
Advertisement.Show(_adUnitId, this);
}
// Implement Load Listener and Show Listener interface methods:
public void OnUnityAdsAdLoaded(string adUnitId)
{
// Optionally execute code if the Ad Unit successfully loads content.
}
public void OnUnityAdsFailedToLoad(string adUnitId, UnityAdsLoadError error, string message)
{
Debug.Log($"Error loading Ad Unit: {adUnitId} - {error.ToString()} - {message}");
// Optionally execute code if the Ad Unit fails to load, such as attempting to try again.
}
public void OnUnityAdsShowFailure(string adUnitId, UnityAdsShowError error, string message)
{
Debug.Log($"Error showing Ad Unit {adUnitId}: {error.ToString()} - {message}");
// Optionally execute code if the Ad Unit fails to show, such as loading another ad.
}
public void OnUnityAdsShowStart(string adUnitId) { }
public void OnUnityAdsShowClick(string adUnitId) { }
public void OnUnityAdsShowComplete(string adUnitId, UnityAdsShowCompletionState showCompletionState) { }
}
Start()でLoadAd()させ、広告を表示したいときにShowAd()を呼び出すと広告が表示される。
RewardedAds
リワード広告を表示するためのスクリプト。リワード広告とは動画を視聴してもらうことでその対価に報酬を与えたりする広告のこと。
参考: https://docs.unity.com/ads/ImplementingRewardedAdsUnity.html
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.Advertisements;
public class RewardedAds : MonoBehaviour, IUnityAdsLoadListener, IUnityAdsShowListener
{
[SerializeField] Button _showAdButton;
[SerializeField] string _androidAdUnitId = "Rewarded_Android";
[SerializeField] string _iOSAdUnitId = "Rewarded_iOS";
string _adUnitId = null; // This will remain null for unsupported platforms
void Awake()
{
// Get the Ad Unit ID for the current platform:
#if UNITY_IOS
_adUnitId = _iOSAdUnitId;
#elif UNITY_ANDROID
_adUnitId = _androidAdUnitId;
#endif
//Disable the button until the ad is ready to show:
_showAdButton.interactable = false;
}
void Start()
{
LoadAd(); //追加
}
// Load content to the Ad Unit:
public void LoadAd()
{
// IMPORTANT! Only load content AFTER initialization (in this example, initialization is handled in a different script).
Debug.Log("Loading Ad: " + _adUnitId);
Advertisement.Load(_adUnitId, this);
}
// If the ad successfully loads, add a listener to the button and enable it:
public void OnUnityAdsAdLoaded(string adUnitId)
{
Debug.Log("Ad Loaded: " + adUnitId);
if (adUnitId.Equals(_adUnitId))
{
// Configure the button to call the ShowAd() method when clicked:
_showAdButton.onClick.AddListener(ShowAd);
// Enable the button for users to click:
_showAdButton.interactable = true;
}
}
// Implement a method to execute when the user clicks the button:
public void ShowAd()
{
// Disable the button:
_showAdButton.interactable = false;
// Then show the ad:
Advertisement.Show(_adUnitId, this);
}
// Implement the Show Listener's OnUnityAdsShowComplete callback method to determine if the user gets a reward:
public void OnUnityAdsShowComplete(string adUnitId, UnityAdsShowCompletionState showCompletionState)
{
if (adUnitId.Equals(_adUnitId) && showCompletionState.Equals(UnityAdsShowCompletionState.COMPLETED))
{
Debug.Log("Unity Ads Rewarded Ad Completed");
// Grant a reward.
// Load another ad:
Advertisement.Load(_adUnitId, this);
}
}
// Implement Load and Show Listener error callbacks:
public void OnUnityAdsFailedToLoad(string adUnitId, UnityAdsLoadError error, string message)
{
Debug.Log($"Error loading Ad Unit {adUnitId}: {error.ToString()} - {message}");
// Use the error details to determine whether to try to load another ad.
}
public void OnUnityAdsShowFailure(string adUnitId, UnityAdsShowError error, string message)
{
Debug.Log($"Error showing Ad Unit {adUnitId}: {error.ToString()} - {message}");
// Use the error details to determine whether to try to load another ad.
}
public void OnUnityAdsShowStart(string adUnitId) { }
public void OnUnityAdsShowClick(string adUnitId) { }
void OnDestroy()
{
// Clean up the button listeners:
_showAdButton.onClick.RemoveAllListeners();
}
}
上記同様Start()でLoadAd()させ、広告を表示したいときにShowAd()を呼び出すと広告が表示される。
BannerAd
バナー広告を表示するためのスクリプト。バナー広告とはアプリの一部に常時表示されるバナー型の広告のこと。
参考: https://docs.unity.com/ads/ImplementingBannerAdsUnity.html
using System.Collections;
using UnityEngine;
using UnityEngine.Advertisements;
public class BannerAd : MonoBehaviour
{
[SerializeField] BannerPosition _bannerPosition = BannerPosition.BOTTOM_CENTER;
[SerializeField] string _androidAdUnitId = "Banner_Android";
[SerializeField] string _iOSAdUnitId = "Banner_iOS";
string _adUnitId = null; // This will remain null for unsupported platforms.
void Start()
{
// Get the Ad Unit ID for the current platform:
#if UNITY_IOS
_adUnitId = _iOSAdUnitId;
#elif UNITY_ANDROID
_adUnitId = _androidAdUnitId;
#endif
SetPosition(); //SetPositionが動作する場合のみ実行させる
LoadBanner();
}
// Implement a method to call when the Load Banner button is clicked:
public void LoadBanner()
{
// もし初期化が完了していなければ待つ
if(Advertisement.isInitialized==false)
{
StartCoroutine(InitializeWhenReady());
}
// Set up options to notify the SDK of load events:
BannerLoadOptions options = new BannerLoadOptions
{
loadCallback = OnBannerLoaded,
errorCallback = OnBannerError
};
// Load the Ad Unit with banner content:
Advertisement.Banner.Load(_adUnitId, options);
}
// Implement code to execute when the loadCallback event triggers:
void OnBannerLoaded()
{
ShowBannerAd();
}
// Implement code to execute when the load errorCallback event triggers:
void OnBannerError(string message)
{
Debug.Log($"Banner Error: {message}");
// Optionally execute additional code, such as attempting to load another ad.
}
// Implement a method to call when the Show Banner button is clicked:
void ShowBannerAd()
{
// もしLoadが完了していなければ待つ
if (Advertisement.Banner.isLoaded == false)
{
StartCoroutine(LoadBannerWhenReady());
}
// Set up options to notify the SDK of show events:
BannerOptions options = new BannerOptions
{
clickCallback = OnBannerClicked,
hideCallback = OnBannerHidden,
showCallback = OnBannerShown
};
// Show the loaded Banner Ad Unit:
Advertisement.Banner.Show(_adUnitId, options);
}
// Implement a method to call when the Hide Banner button is clicked:
void HideBannerAd()
{
// Hide the banner:
Advertisement.Banner.Hide();
}
void OnBannerClicked() { }
void OnBannerShown() { }
void OnBannerHidden() { }
public void SetPosition()
{
Advertisement.Banner.SetPosition(_bannerPosition);
}
IEnumerator InitializeWhenReady()
{
while (Advertisement.isInitialized == false)
{
yield return new WaitForSeconds(3.0f);
}
LoadBanner();
}
IEnumerator LoadBannerWhenReady()
{
if(Advertisement.Banner.isLoaded==false)
{
yield return new WaitForSeconds(3.0f);
}
LoadBanner();
}
}
Unityのドキュメント例ではボタンを使うことで読み込みとその後の動作をコントロールするようにしていましたが、バナー広告はアプリ起動後に表示できる準備が整い次第すぐに表示して欲しかったので、読み込みが完了しているかを確認し、完了していなれば少し待ってからもう一度実行するようにしました。待ち時間がないと読み込みが完了していないにもかかわらず次の関数が実行されてしまうため、広告がうまく表示できませんでした。
Advertisement.Banner.SetPosition(_bannerPosition)について
バナー広告の表示位置を決めるAdvertisement.Banner.SetPosition(_bannerPosition)ですが、Android studioで動作確認すると処理が止まってしまうという謎現象が発生しました。同様に処理が止まるようであれば、SetPosition関数の呼び出しを行わないようにしてください。SetPositionしない場合、バナー広告は常にアプリ左上に表示されます。
スクリプトをアタッチしてテスト
作成したスクリプトをアタッチし、テストする。
AdsInitializerについて
AdsInitializerはシーン内のどこかに一つアタッチされていれば良い。今回はバナー広告用のGameObjectにアタッチしている。
BannerAd
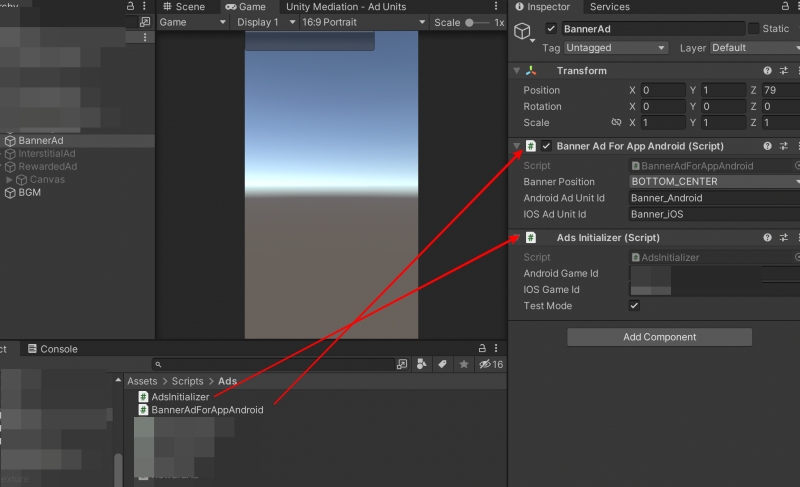
空のGameObjectを作成し、AdsInitializerとBannerAdをアタッチする。(BannerAdのファイル名が違いますが気にしないでください)
Unit id はそのままで良いが、確認したい場合はUnity Gaming ServicesのAd Unitsで確認できる(GameIDを確認した画面で確認できる)。
AdsInitializerにはGameIDを入力する。GameIDの取得の仕方は以下で解説。
ProjectSettingsでtest modeをONにしているのでチェックしなくてもテストモードで起動すると思うが、念のためチェックしておく。
テストプレイをすると、バナー広告が表示される。SetPositionを実行していない場合は、左上に広告が表示される。
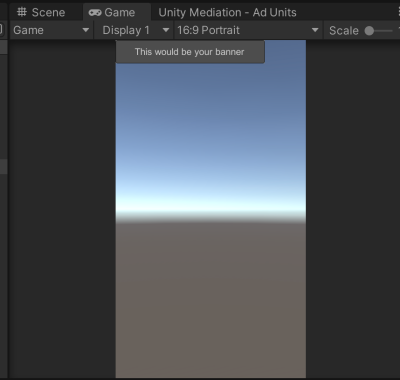
InterstitialAd
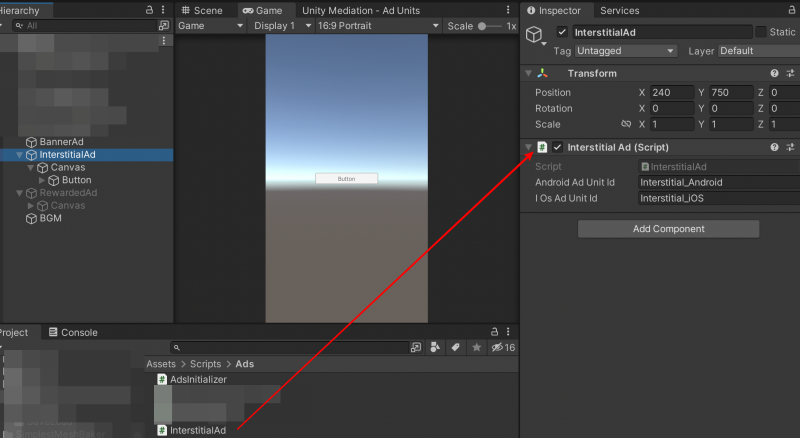
空のGameObjectを作成し、InterstitialAdをアタッチする。※AdsInitializerは他のGameObjectにアタッチされていること。
Unit id はそのままで良いが、確認したい場合はUnity Gaming ServicesのAd Unitsで確認できる(GameIDを確認した画面で確認できる)。
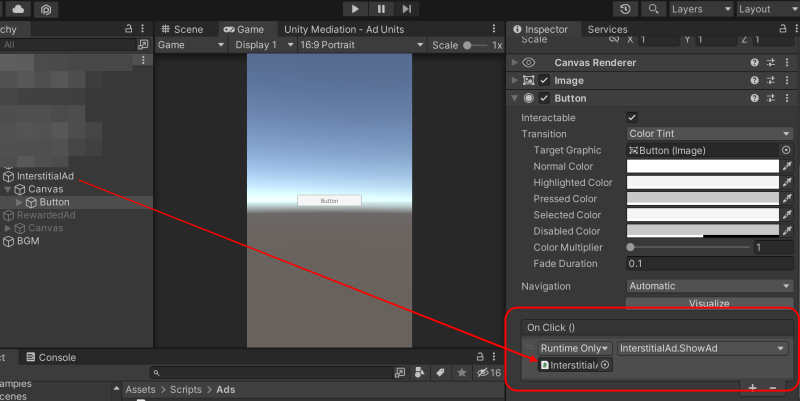
Buttonオブジェクトを作成し、InterstitialAdをアタッチしているGameObjectをButtonのOnClick()にアタッチし、ShowAdを実行するように設定する。
テストプレイを実行し、ボタンをクリックすると広告が表示される。
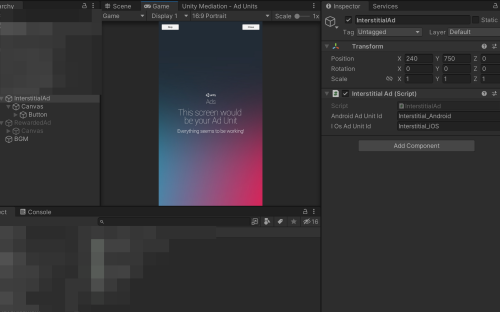
RewardedAds
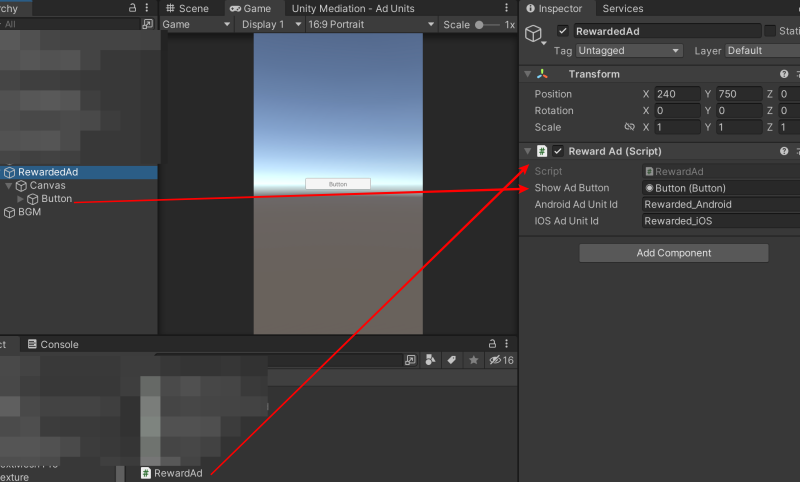
空のGameObjectを作成し、RewardAdをアタッチする。※AdsInitializerは他のGameObjectにアタッチされていること。
Unit id はそのままで良いが、確認したい場合はUnity Gaming ServicesのAd Unitsで確認できる(GameIDを確認した画面で確認できる)。
Buttonオブジェクトを作成し、RewardAdにアタッチする。
テストプレイをし、Buttonを押すと広告が表示される。
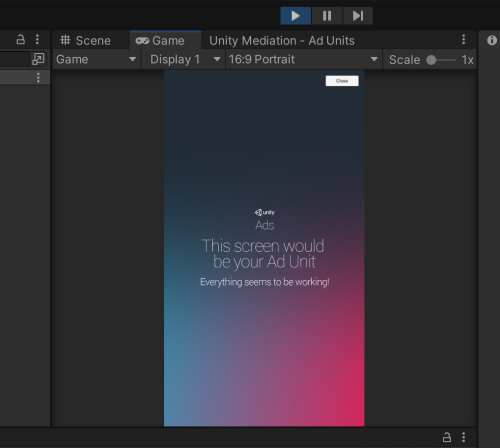
インターシティシャル広告と違い、左上にskipボタンが表示されない。
おまけ
上記の設定でビルドし、Android Studioで動作テストをしたところ、広告は表示されました。インターシティシャル広告とリワード広告はUnityのテストプレイでは静止画ですが、Android Studioではきちんと動画が流れます。バナー広告もUnityAdsのリンクと画像が表示されます。
バナー広告はSetPositionで動作が止まり、広告が表示されないという謎の現象が起こる可能性があるので注意してください。
コメント